Say you want to develop an app where users can post status / tweet (like Facebook / Twitter) and other users can view it, how do you start? You might have gotten suggestions that you might need Firebase database or a node.js powered ‘backend’ , what does these mean? What keywords should we search in Google to get more information on this?
Let’s use Twitter app as an example, say you posted a new tweet from the app, the app will then send the tweet to the Twitter server. Then your follower will ask Twitter server for new tweets and retrieve them.
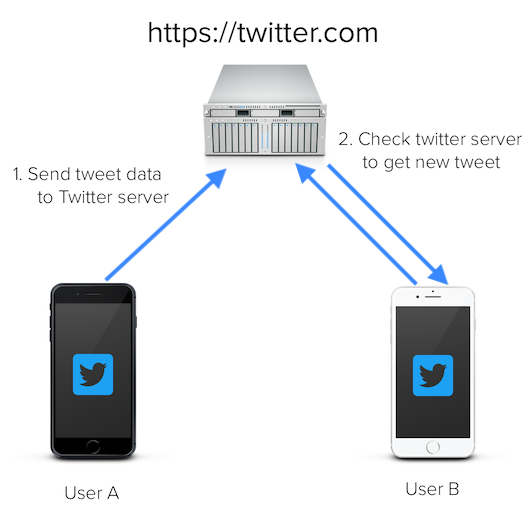
The server is needed as it stores your tweet data, and is publicly accessible by others devices. Without a server, your tweet data can only exist inside your device and other people would not be able to see it.
Similar to the app, server also runs code to handle the request from the app, and save/load the data to/from the database. Some of the most popular server side language includes PHP, Python, Java, Ruby etc. As developing server side app from scratch takes a lot of effort, there’s frameworks specific to each language that comes with prebuilt feature, such as Ruby on Rails (framework for Ruby), Laravel (framework for PHP) etc, you can think of these frameworks like the libraries / Cocoapods you used for your iOS projects.
When you hear people mentioning backend, it usually means the server side. It’s called ‘backend’ because user doesn’t see this part, as they only see the UI of the app. Servers are computer as well, it has the same structure of hard disk / SSD, memory (RAM), CPU compared to our computer, it can be beefier or less powerful than our computer (Raspberry pi!). As long as it serve a response to the requesting device, it can be called as server.
The opposite of is frontend (client side), this is what the user sees, eg: iOS app UI, or the HTML webpage shown in your web browser.
Usually the server stores data in a database, some example of database management system : MySQL, PostgreSQL, etc. Most database uses a relational model that utilize multiple tables :
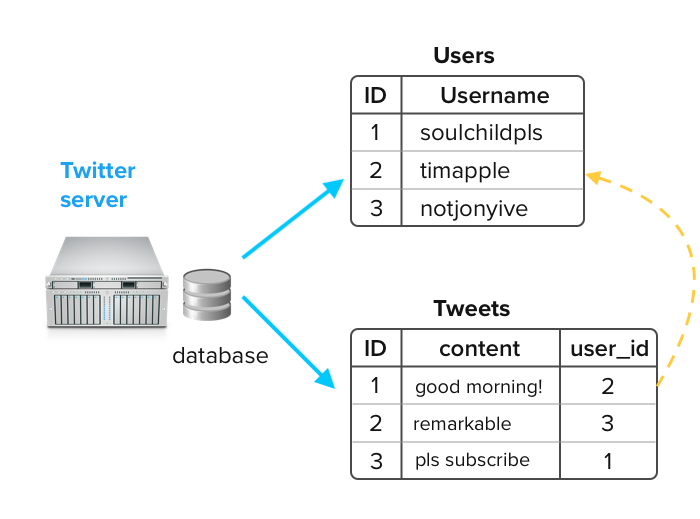
Usually mobile apps don’t connect to the database and manipulate the data directly (eg: inserting new row, modify existing row), as this might expose potential security risk (some malicious user might modify the app to perform unauthorized data modification on your database).
Most apps usually communicate to the server using an API (Application Programming Interface), also known as API endpoint. Some of the Twitter API endpoints looks like this :
https://api.twitter.com/1.1/statuses/update
https://api.twitter.com/1.1/statuses/destroy/:id.json
https://api.twitter.com/1.1/statuses/retweet/:id
These looks like a URL you type in your web browser and yes they are actually a URL!
When you type in a URL in your web browser (eg: Safari, Chrome, etc) , your web browser will send a HTTP GET request to the URL.
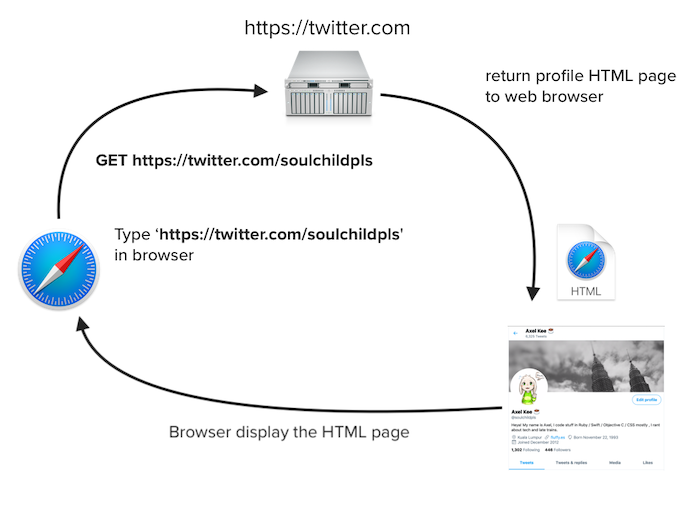
To send a new tweet to the server, the Twitter app probably will call the API like this :
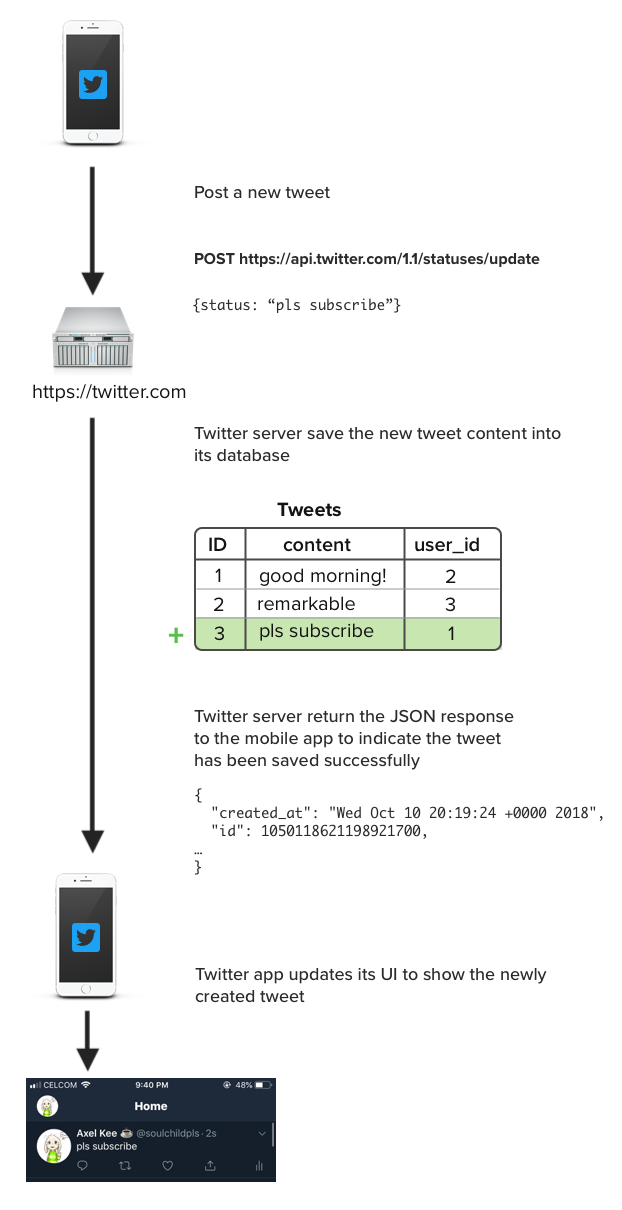
The GET and POST request are two of the HTTP method. HTTP is a communication protocol that is widely used in the internet for communication between devices, one of the most important feature of it is that it is stateless and depend on request-response mechanism, meaning to get a response from another device, we must first send a request.
For devices using HTTP protocol to communicate, the communication starts with a HTTP request. There’s multiple type of HTTP request like GET (This is what your web browser will call when visiting a URL), POST (When you submit form, eg: registration/login form, new tweet form etc), PUT, PATCH, DELETE etc.
HTTP Request is essentially text that follows a specified format. Here’s how a raw POST HTTP Request looks like:
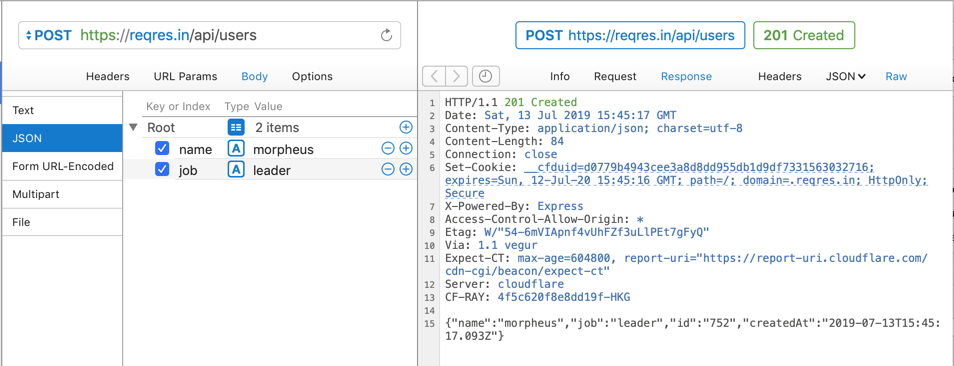
There’s communication protocol other than HTTP such as socket, which keeps an open connection between devices so that the server can push data directly to the client without client requesting it.
Sending HTTP request and parsing response in iOS
Apple has provided us convenient tools to send HTTP request and parse the JSON response retrieved.
To send a HTTP request from the iOS app to server, we can use URLSession class, you can read more on how to use it here .
JSON (JavaScript Object Notation) is a type of data format, which can contain dictionary, array, boolean, null, string and number. It is enclosed with a curly bracket, eg:
{ "name":"John", "age":30, "products": ["iPhone X", "iPad Pro"] }
You can think of JSON as a formatted string that follows the format above.
Most server API usually returns JSON object (as String), to turn them into Swift class / struct, we can use the handy Decodable protocol. You can read more on how to use it here.
CloudKit and Firebase
Up until now, we have been using Twitter server as an example of backend, we can easily swap this with CloudKit or Firebase as they also provide an API for our app to communicate with, and also have a database that stores data.
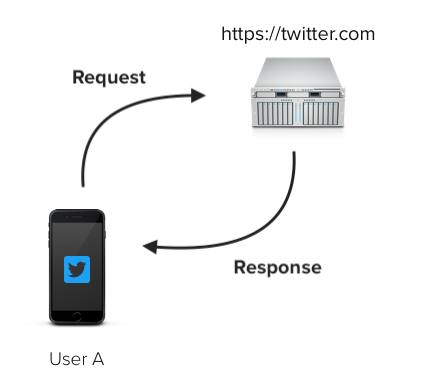
CloudKit (iCloud) :
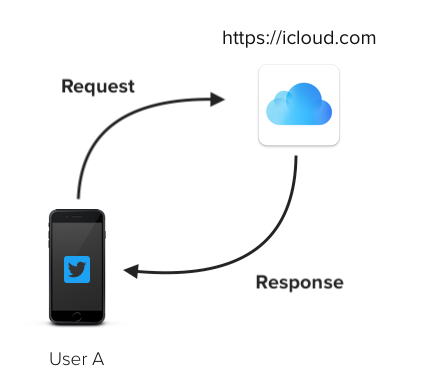
Firebase :
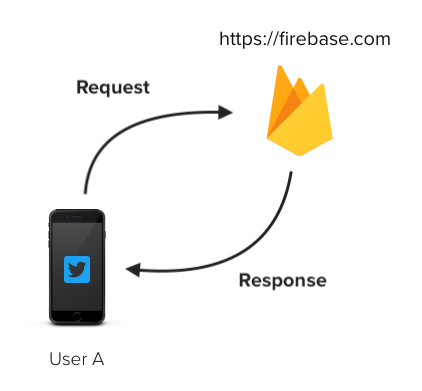
Firebase database structure
One thing to note that the database structure of Firebase is different than the relational model we mentioned earlier.
In a relational database model, users and tweets are stored in tables, and we can check which user wrote the tweet by referencing the user id in the tweet table.
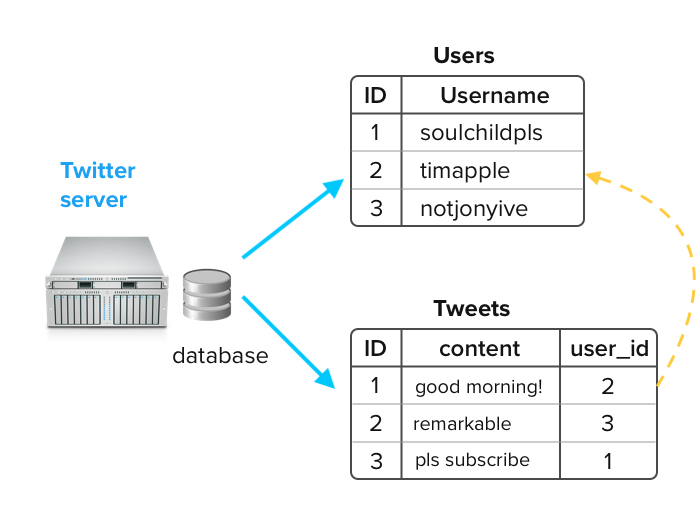
Firebase database doesn’t use table structure, instead, it save everything as JSON structure. So for a user list, Firebase saves it like this :
{
"users": {
"soulchildpls": {
"display_name": "Axel Kee",
},
"tim_cook": {
"display_name": "Tim Cook",
},
"JonyIveParody": {
"display_name": "Jony Ive"
}
}
}
soulchildpls , timcook , JonyIveParody are the unique identifier (like the 1,2,3 ID in the relational table), and each of them has the property “displayname”
To save tweets and users into Firebase, the structure might look like this :
{
"users": {
"soulchildpls": {
"display_name": "Axel Kee",
},
"tim_cook": {
"display_name": "Tim Cook",
},
"JonyIveParody": {
"display_name": "Jony Ive"
}
},
"tweets": {
"one": {
"message": "Don't just follow your dream, follow my twitter too",
"author": "soulchildpls".
},
"two": {
"message": "The best iPhone yyyyettt",
"author": "tim_cook"
},
}
We now have added the “tweets” key into the Firebase JSON structure. The “one” and “two” are the unique identifier for the tweets (like the 1,2,3 ID in the relational table), and the “author” refers to the unique identifier of the users.
You can read more about the Firebase JSON tree structure here.
What backend should I use?
Chances are, your app idea might require a backend to store user data. For example, a to-do list that can be synced across different devices, or a quiz question where a teacher can share to his/her students, or a social networking app where user can share his/her post for others to see.
If you app doesn’t require data to be stored outside of the device (everything is stored just inside the user phone and no syncing, if user delete the app, all the data will be gone as well), you probably don’t need a backend.
It can be daunting to build your own backend server program and putting them online. If you have no prior backend programming experience and just starting iOS development, I recommend using Apple’s CloudKit or Google’s Firebase.
The pros of using CloudKit as backend is that it is first party (supported and maintained by Apple) and it integrates with iOS seamlessly, the huge downside is that CloudKit database is iOS-only, if you plan to build an Android version of your app in the future, then you are out of luck 😅. CloudKit has a very generous quota for their free plan, its very hard to use over their quota, so you can treat it as a free solution!
The pros of using Firebase is that it supports both iOS and Android platform, you can use the same backend for both platform. The cons is that you will need to install it as a dependency (using Cocoapods), and you will need to learn the database structure (JSON tree)of Firebase. Firebase has a free plan to help you get started, but be careful once you have input your card details into Firebase payment, if your Firebase queries / usage are not optimized, you might end up with a 5 figures bill in a month.
Want to start learning how to write your own backend code?
You get advice like “just google it! there’s tons of tutorial on Google!” , but the problem is that there’s too much resources / information on Google! A simple search for getting started with backend code can quickly turn into setting up various stuff like PostgreSQL / framework / server etc on your computer.
I have written a quick concise guide on how to write a basic backend code (using Ruby + Sinatra) with API that your iOS app can call, and return a JSON response. The guide also include how to put your backend code online so your user using the app can access it!
You can get the guide here :
https://drive.google.com/file/d/1iF9_6iO1Lrjx_-6CpvIHXVsNYTblhasm/view?usp=sharing